Jan 02, 2014 Python interface to MySQL. MySQLdb is an interface to the popular MySQL database server for Python. The design goals are: Compliance with Python database API version 2.0. EASME - Executive Agency for SMEs.
The command-line interface (CLI) tools for Entity Framework Core perform design-time development tasks. For example, they create migrations, apply migrations, and generate code for a model based on an existing database. The commands are an extension to the cross-platform dotnet command, which is part of the .NET Core SDK. These tools work with .NET Core projects.
If you're using Visual Studio, we recommend the Package Manager Console tools instead:
- They automatically work with the current project selected in the Package Manager Console without requiring that you manually switch directories.
- They automatically open files generated by a command after the command is completed.
Installing the tools
The installation procedure depends on project type and version:
- EF Core 3.x
- ASP.NET Core version 2.1 and later
- EF Core 2.x
- EF Core 1.x
EF Core 3.x
dotnet ef
must be installed as a global or local tool. Most developers will installdotnet ef
as a global tool with the following command:Need to straighten your photo?
You can also use
dotnet ef
as local tool. To use it as a local tool, restore the dependencies of a project that declares it as a tooling dependency using a tool manifest file.Install the .NET Core SDK.
Install the latest
Microsoft.EntityFrameworkCore.Design
package.
ASP.NET Core 2.1+
Install the current .NET Core SDK. The SDK has to be installed even if you have the latest version of Visual Studio 2017.
This is all that is needed for ASP.NET Core 2.1+ because the
Microsoft.EntityFrameworkCore.Design
package is included in the Microsoft.AspNetCore.App metapackage.
EF Core 2.x (not ASP.NET Core)
The dotnet ef
commands are included in the .NET Core SDK, but to enable the commands you have to install the Microsoft.EntityFrameworkCore.Design
package.
Install the current .NET Core SDK. The SDK has to be installed even if you have the latest version of Visual Studio.
Install the latest stable
Microsoft.EntityFrameworkCore.Design
package.
EF Core 1.x
Install the .NET Core SDK version 2.1.200. Later versions are not compatible with CLI tools for EF Core 1.0 and 1.1.
Configure the application to use the 2.1.200 SDK version by modifying its global.json file. This file is normally included in the solution directory (one above the project).
Edit the project file and add
Microsoft.EntityFrameworkCore.Tools.DotNet
as aDotNetCliToolReference
item. Specify the latest 1.x version, for example: 1.1.6. See the project file example at the end of this section.Install the latest 1.x version of the
Microsoft.EntityFrameworkCore.Design
package, for example:With both package references added, the project file looks something like this:
A package reference with
PrivateAssets='All'
isn't exposed to projects that reference this project. This restriction is especially useful for packages that are typically only used during development.
Verify installation
Run the following commands to verify that EF Core CLI tools are correctly installed:
The output from the command identifies the version of the tools in use:
Using the tools
Before using the tools, you might have to create a startup project or set the environment.
Target project and startup project
The commands refer to a project and a startup project.
The project is also known as the target project because it's where the commands add or remove files. By default, the project in the current directory is the target project. You can specify a different project as target project by using the
option.--project
The startup project is the one that the tools build and run. The tools have to execute application code at design time to get information about the project, such as the database connection string and the configuration of the model. By default, the project in the current directory is the startup project. You can specify a different project as startup project by using the
option.--startup-project
The startup project and target project are often the same project. A typical scenario where they are separate projects is when:
- The EF Core context and entity classes are in a .NET Core class library.
- A .NET Core console app or web app references the class library.
It's also possible to put migrations code in a class library separate from the EF Core context.
Other target frameworks
The CLI tools work with .NET Core projects and .NET Framework projects. Apps that have the EF Core model in a .NET Standard class library might not have a .NET Core or .NET Framework project. For example, this is true of Xamarin and Universal Windows Platform apps. In such cases, you can create a .NET Core console app project whose only purpose is to act as startup project for the tools. The project can be a dummy project with no real code — it is only needed to provide a target for the tooling.
Why is a dummy project required? As mentioned earlier, the tools have to execute application code at design time. To do that, they need to use the .NET Core runtime. When the EF Core model is in a project that targets .NET Core or .NET Framework, the EF Core tools borrow the runtime from the project. They can't do that if the EF Core model is in a .NET Standard class library. The .NET Standard is not an actual .NET implementation; it's a specification of a set of APIs that .NET implementations must support. Therefore .NET Standard is not sufficient for the EF Core tools to execute application code. The dummy project you create to use as startup project provides a concrete target platform into which the tools can load the .NET Standard class library.
ASP.NET Core environment
To specify the environment for ASP.NET Core projects, set the ASPNETCORE_ENVIRONMENT environment variable before running commands.
Common options
Option | Description | |
---|---|---|
--json | Show JSON output. | |
-c | --context <DBCONTEXT> | The DbContext class to use. Class name only or fully qualified with namespaces. If this option is omitted, EF Core will find the context class. If there are multiple context classes, this option is required. |
-p | --project <PROJECT> | Relative path to the project folder of the target project. Default value is the current folder. |
-s | --startup-project <PROJECT> | Relative path to the project folder of the startup project. Default value is the current folder. |
--framework <FRAMEWORK> | The Target Framework Moniker for the target framework. Use when the project file specifies multiple target frameworks, and you want to select one of them. | |
--configuration <CONFIGURATION> | The build configuration, for example: Debug or Release . | |
--runtime <IDENTIFIER> | The identifier of the target runtime to restore packages for. For a list of Runtime Identifiers (RIDs), see the RID catalog. | |
-h | --help | Show help information. |
-v | --verbose | Show verbose output. |
--no-color | Don't colorize output. | |
--prefix-output | Prefix output with level. |
dotnet ef database drop
Drops the database.
Options:
Option | Description | |
---|---|---|
-f | --force | Don't confirm. |
--dry-run | Show which database would be dropped, but don't drop it. |
dotnet ef database update
Updates the database to the last migration or to a specified migration.
Arguments:
Argument | Description |
---|---|
<MIGRATION> | The target migration. Migrations may be identified by name or by ID. The number 0 is a special case that means before the first migration and causes all migrations to be reverted. If no migration is specified, the command defaults to the last migration. |
The following examples update the database to a specified migration. The first uses the migration name and the second uses the migration ID:
dotnet ef dbcontext info
Gets information about a DbContext
type.
dotnet ef dbcontext list
Lists available DbContext
types.
dotnet ef dbcontext scaffold
Generates code for a DbContext
and entity types for a database. In order for this command to generate an entity type, the database table must have a primary key.
Arguments:
Argument | Description |
---|---|
<CONNECTION> | The connection string to the database. For ASP.NET Core 2.x projects, the value can be name=<name of connection string>. In that case the name comes from the configuration sources that are set up for the project. |
<PROVIDER> | The provider to use. Typically this is the name of the NuGet package, for example: Microsoft.EntityFrameworkCore.SqlServer . |
Options:
Option | Description | |
---|---|---|
--data-annotations | Use attributes to configure the model (where possible). If this option is omitted, only the fluent API is used. | |
-c | --context <NAME> | The name of the DbContext class to generate. |
--context-dir <PATH> | The directory to put the DbContext class file in. Paths are relative to the project directory. Namespaces are derived from the folder names. | |
-f | --force | Overwrite existing files. |
-o | --output-dir <PATH> | The directory to put entity class files in. Paths are relative to the project directory. |
--schema <SCHEMA_NAME>.. | The schemas of tables to generate entity types for. To specify multiple schemas, repeat --schema for each one. If this option is omitted, all schemas are included. | |
-t | --table <TABLE_NAME> .. | The tables to generate entity types for. To specify multiple tables, repeat -t or --table for each one. If this option is omitted, all tables are included. |
--use-database-names | Use table and column names exactly as they appear in the database. If this option is omitted, database names are changed to more closely conform to C# name style conventions. |
The following example scaffolds all schemas and tables and puts the new files in the Models folder.
The following example scaffolds only selected tables and creates the context in a separate folder with a specified name:
dotnet ef migrations add
Adds a new migration.
Arguments:
Projects Db 1.2 3
Argument | Description |
---|---|
<NAME> | The name of the migration. |
Options:
Option | Description | |
---|---|---|
-o | --output-dir <PATH> | The directory (and sub-namespace) to use. Paths are relative to the project directory. Defaults to 'Migrations'. |
dotnet ef migrations list
Lists available migrations.
dotnet ef migrations remove
Removes the last migration (rolls back the code changes that were done for the migration).
Options:
Option | Description | |
---|---|---|
-f | --force | Revert the migration (roll back the changes that were applied to the database). |
dotnet ef migrations script
Generates a SQL script from migrations.
Arguments:
Argument | Description |
---|---|
<FROM> | The starting migration. Migrations may be identified by name or by ID. The number 0 is a special case that means before the first migration. Defaults to 0. |
<TO> | The ending migration. Defaults to the last migration. |
Options:
Option | Description | |
---|---|---|
-o | --output <FILE> | The file to write the script to. |
-i | --idempotent | Generate a script that can be used on a database at any migration. |
The following example creates a script for the InitialCreate migration:
The following example creates a script for all migrations after the InitialCreate migration.
Additional resources
Latest versionReleased:
Microsoft Azure CosmosDB Table Client Library for Python
Project description
Microsoft Azure CosmosDB Table SDK for Python
This project provides a client library in Python that makes it easy toconsume Microsoft Azure CosmosDB Table services. For documentation please seethe Microsoft Azure Python Developer Center and our API Reference Page.
If you are looking for the Service Bus or Azure Managementlibraries, please visithttps://github.com/Azure/azure-sdk-for-python.
Compatibility
IMPORTANT: If you have an earlier version of the azure package(version < 1.0), you should uninstall it before installing this package.
You can check the version using pip:
If you see azure0.11.0 (or any version below 1.0), uninstall it first then install it again:
Features
- Table
- Create/Read/Update/Delete Tables
- Create/Read/Update/Delete Entities
- Batch operations
- Advanced Table Operations
Getting Started
Download
Option 1: Via PyPi
To install via the Python Package Index (PyPI), type:
Option 2: Source Via Git
To get the source code of the SDK via git just type:
Option 3: Source Zip
Download a zip of the code via GitHub or PyPi. Then, type:
Minimum Requirements
- Python 2.7, 3.3, 3.4, 3.5, or 3.6.
- See setup.py for dependencies
Usage
To use this SDK to call Microsoft Azure storage services, you need tofirst create an account.
Code Sample
See the samples directory for table usage samples.
Need Help?
Be sure to check out the Microsoft Azure Developer Forums on MSDN orthe Developer Forums on Stack Overflow if you have trouble with theprovided code.
Projects Db 1.2 Minecraft
Contribute Code or Provide Feedback
If you would like to become an active contributor to this project, pleasefollow the instructions provided in Azure Projects ContributionGuidelines. You can find more details for contributing in the CONTRIBUTING.md doc.
Projects Db 1.2 Online
If you encounter any bugs with the library, please file an issue in theIssues section of the project.
Learn More
Release historyRelease notifications
1.0.6
1.0.5
1.0.4
1.0.3
1.0.2
1.0.1
1.0.0
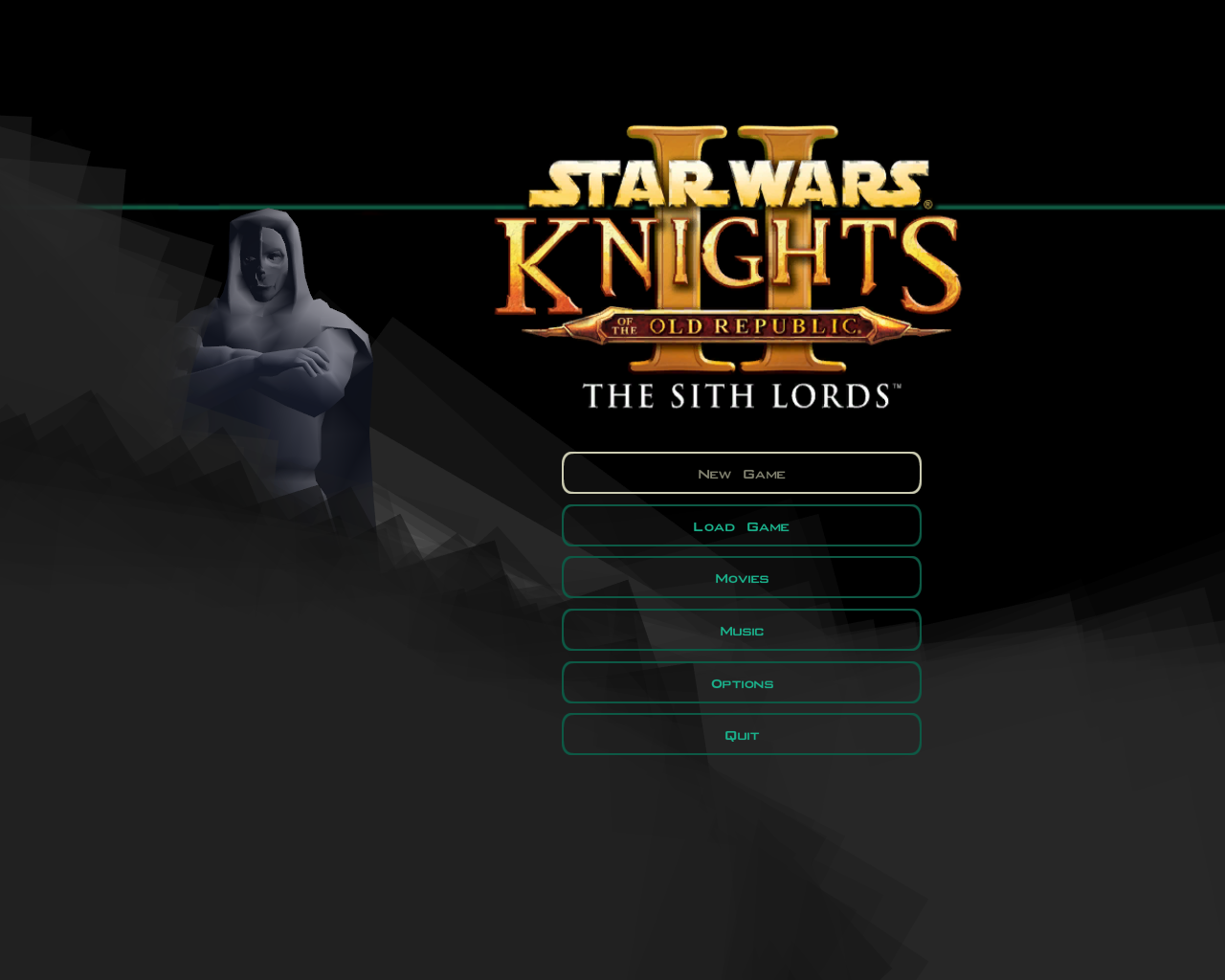
0.37.2
0.37.1
0.37.0
Download files
Download the file for your platform. If you're not sure which to choose, learn more about installing packages.
Filename, size | File type | Python version | Upload date | Hashes |
---|---|---|---|---|
Filename, size azure_cosmosdb_table-1.0.6-py2.py3-none-any.whl (125.6 kB) | File type Wheel | Python version py2.py3 | Upload date | Hashes |
Filename, size azure-cosmosdb-table-1.0.6.tar.gz (93.5 kB) | File type Source | Python version None | Upload date | Hashes |
Hashes for azure_cosmosdb_table-1.0.6-py2.py3-none-any.whl
Algorithm | Hash digest |
---|---|
SHA256 | ee525233d6c8c016526593bf28f8a374275cfe204a00c41134b83a1736f7b5f7 |
MD5 | a05ce10da63f08ce7070787c1033d3cb |
BLAKE2-256 | f0e415a59108883cc47460b1475aeac935e2d975b5def42f2c0a8b8fd48b3304 |
Hashes for azure-cosmosdb-table-1.0.6.tar.gz
Algorithm | Hash digest |
---|---|
SHA256 | 5f061d2ab8dcf2f0b4e965d5976e7b7aeb1247ea896911f0e1d29092aaaa29c7 |
MD5 | 3f8897ba3ca71487f91ff352c7a1739f |
BLAKE2-256 | 447fed12909a12adddd9bc7b00f5d290af1e28b1d55f4e92f2114302ad309445 |